Introduction: Anime.js JavaScript Library
If you’re venturing into the world of web animations, you’ve likely come across Anime.js — a powerful and lightweight JavaScript animation library. Anime.js is known for its ease of use and flexibility, enabling developers to create stunning animations with minimal code. In our previous article, we discussed Anime.js in detail, covering its core concepts, syntax, and usage examples.
However, while Anime.js is user-friendly, beginners often encounter a variety of issues that can hinder their animation workflow. These issues may result in broken animations, unexpected behavior, or performance bottlenecks. In this post, we’ll dive deep into the 25 most common Anime.js problems, explain why they occur, and offer practical solutions with code examples to help you avoid or fix them.
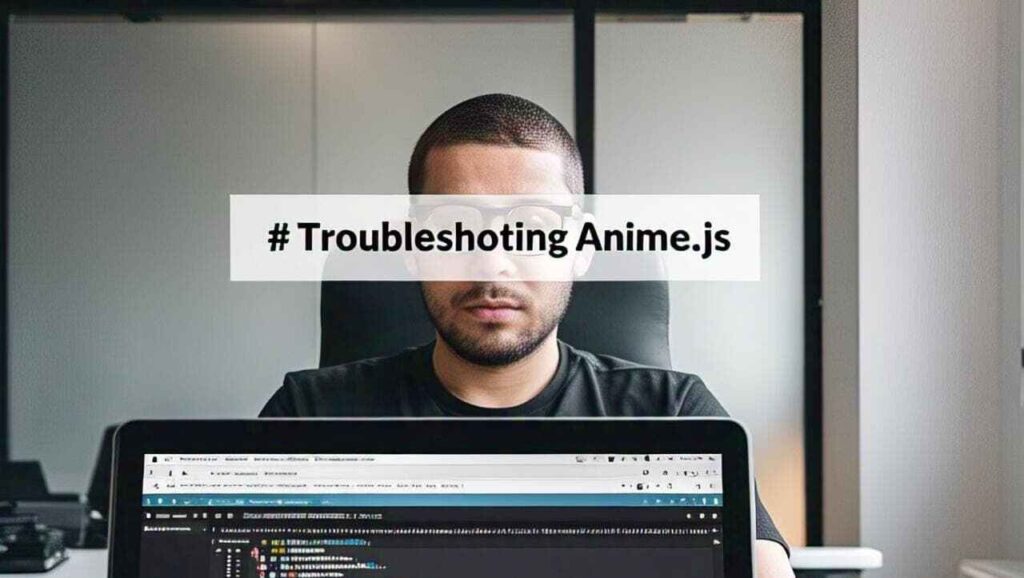
Whether you’re just starting or already using Anime.js in your projects, this guide will help you debug and optimize your animations like a pro.
Most Common Issues And Mistake In Anime.js
1.Incorrect or Missing Anime.js Script Link
Issue: The Anime.js script is not correctly linked or is missing entirely.
Why It Happens: Beginners often forget to include the Anime.js CDN or reference the wrong file path.
Solution: Always ensure the script is properly included in your HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/animejs/3.2.1/anime.min.js"></script>
Make sure it is included before your custom scripts that use Anime.js.
2.Using Invalid or Non-existent CSS Selectors
Issue: The target selector passed to Anime.js doesn’t match any element.
Why It Happens: A typo in the selector or the element isn’t present in the DOM.
Solution: Double-check selectors and make sure elements exist when the animation runs:
anime({
targets: '.box',
translateX: 250
});
Use console.log(document.querySelector(‘.box’)) to verify existence.
3.Missing Units in Property Values
Issue: Not using units like px or deg when required.
Why It Happens: Anime.js requires units for certain CSS properties.
Solution: Add units manually:
anime({
targets: '.box',
rotate: '180deg',
width: '200px'
});
4.Animating Non-Animatable CSS Properties
Issue: Trying to animate properties that CSS doesn’t allow to animate.
Solution: Only animate supported properties such as:
transform
opacity
backgroundColor
width/height (with caution)
Refer to MDN Animatable Properties.
5. Animations Not Triggering on Page Load
Why It Happens: Scripts execute before the DOM is fully loaded.
Solution: Use window.onload or place script at the bottom:
window.onload = function() {
anime({ targets: '.box', opacity: 1 });
};
6. Multiple anime() Calls Not Syncing Properly
Issue: Animations running out of sync.
Solution: Use Anime.js timelines:
let tl = anime.timeline();
ttl.add({ targets: '.box', translateX: 100 })
.add({ targets: '.circle', opacity: 0.5 });
7. Ignoring the Anime.js Timeline Feature
Why It Matters: Timelines allow sequencing multiple animations.
Solution: Utilize anime.timeline() for better control and cleaner code.
8. Misusing loop, autoplay, or direction Options
Issue: Animations behave unexpectedly.
Solution: Understand what each option does:
anime({
targets: '.box',
translateX: 250,
loop: true,
autoplay: false,
direction: 'alternate'
});
9. Overwriting Styles with Inline CSS
Issue: Styles set by Anime.js are overridden.
Solution: Avoid using inline styles or override them after animation.
10. Animations Running Before DOM is Ready
Solution: Wrap code in:
document.addEventListener("DOMContentLoaded", function() {
// animation code
});
11.Forgetting to Use Callback Functions
Tip: Use begin, complete, etc. to hook into animation lifecycle:
anime({
targets: '.box',
complete: () => console.log('Done!')
});
12. Setting Targets to null or Empty NodeList
Issue: Target element is not found.
Fix: Ensure valid selector or DOM existence:
if (document.querySelector('.box')) {
anime({ targets: '.box', translateX: 200 });
}
13. Using Unsupported Easing Functions
Solution: Refer to Anime.js docs for supported easing types like easeInOutQuad, spring(1, 80, 10, 0).
14. Not Understanding Animation Duration Properly
Issue: Wrong time unit or misunderstood value.
Tip: Duration is always in milliseconds:
anime({ duration: 1000 }); // 1 second
15. Animating Too Many Elements at Once
Problem: Leads to performance drops.
Fix: Limit targets or batch animations using stagger.
anime({
targets: '.box',
translateX: 100,
delay: anime.stagger(100)
});
16. Ignoring Browser Compatibility
Tip: Use progressive enhancement. Check for animation support using feature detection.
17. Forgetting Quotes in Keyframes
Fix: Strings must be quoted:
keyframes: [
{ translateX: '100px' },
{ translateY: '50px' }
]
18. Applying Animation on Display: none Elements
Fix: Use visibility hidden or opacity 0 instead:
.box {
visibility: hidden;
}
19. Combining Anime.js with CSS Transitions Improperly
Problem: Results in animation conflict.
Fix: Use one method consistently.
20. Assuming Anime.js Will Handle Element Visibility
Reality: It only handles properties you define.
Fix: Add opacity or visibility explicitly in animation.
21. Not Resetting Styles Before Re-running Animations
Tip: Reset properties manually before triggering again.
22. Confusing Between Object vs Array Animation Targets
Clarification:
anime({ targets: ['.box1', '.box2'] });
23. Misunderstanding the Role of delay in Sequences
Tip: delay helps stagger animations; useful in list UI animations.
24. Not Using Developer Tools to Debug Issues
Fix: Use browser DevTools (console, inspector, breakpoints) for real-time debugging.
25. Trying to Animate Elements That Don’t Exist Yet
Fix: Use MutationObserver or delay code execution until DOM elements are ready.
Suggestions Abut Anime.js Issues:
- Always test animations in multiple browsers.
- Use anime.timeline() for complex sequences.
- Keep animations short and meaningful.
- Use semantic class names.
- Avoid animating non-visible elements.
Conclusion:
Anime.js is a fantastic tool for creating smooth, elegant, and interactive web animations, but even seasoned developers can fall into these common pitfalls. Understanding the root causes and learning how to address them will make your code more reliable and your animations more impactful. If you’re facing issues with Anime.js, revisit these 25 common problems, and you’ll find a solution that helps you move forward confidently.
For a detailed understanding of Anime.js from the ground up, don’t forget to check out our previous guide on how to get started with Anime.js.
FAQs’
Anime.js cannot animate elements with display: none because they are not rendered in the DOM. Instead, set the element’s visibility to hidden and opacity to 0, then animate the opacity and set visibility to visible during the animation.
To enhance performance on devices with limited resources, reduce the number of simultaneous animations, use hardware-accelerated properties like transform and opacity, and consider lowering the global frame rate using engine.fps = 30;.
This issue often arises when animation properties are not reset. Always reinitialize the anime() instance or reset styles before retriggering the animation to ensure it plays correctly each time.
A: This error typically occurs when Anime.js is not properly imported in a module-based setup. Install Anime.js via npm and import it using import anime from ‘animejs/lib/anime.es.js’;. If you’re using a
Ensure that the DOM is fully loaded before executing your animation code. Wrap your code inside a DOMContentLoaded event listener. Also, verify that the target elements exist and are not set to display: none, as Anime.js cannot animate elements that are not rendered.
Read Our Others Post’s
Anime.js Animation Library Explained in 60 second’s
Share With FriendsIntroduction of Anime.js In the world of web development, animation plays a huge role in making websites interactive […]
19 Common CSS Grid and Flexbox Issues You Need to Fix Now!
Share With FriendsIntroduction In today’s web development landscape, CSS Grid and Flexbox are essential tools that every developer must master. […]
Stop Using CSS Grid and Flexbox Wrong! Try These Tips
Modern web development relies heavily on responsive and flexible layouts to provide a seamless user experience across devices. CSS Grid and Flexbox are two of the most powerful tools for web designers and developers.
Good